Project-Based Python Learning Course
Welcome to the Project-Based Python Learning Course! This repository, curated by dsj7419, is designed to provide a hands-on and engaging approach to learning Python through practical projects. This is a constant work in progress, and is evolving quickly!
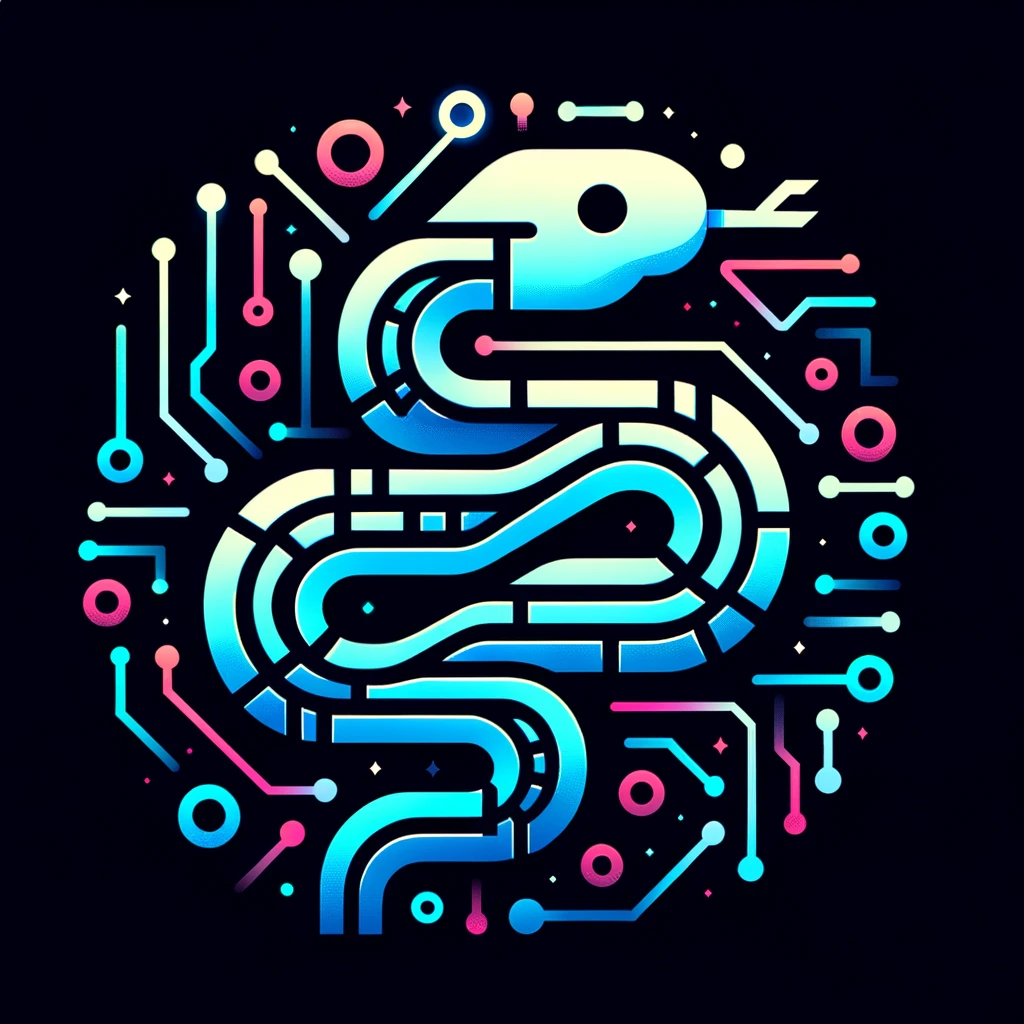
About The Course
This course is structured into different modules, each focusing on a particular topic in Python and associated with a project to provide a practical application of the concepts learned.
How to Use This Repository
- Learners: Navigate through the modules, read the concepts, and work on projects on the course website. Each lesson is available as a separate page, and quizzes are integrated into the web interface for a seamless learning experience.
- Contributors: Feel free to improve existing content, add new projects, or enhance documentation by interacting with the GitHub repository.
Getting Started
Start your Python journey here by setting up your development environment and working on the first project!
Course Structure
Beginning Python
- Getting Started
- Environment Setup, Writing a Python Script
- Project: Hello, World! & Magic 8-Ball Simulator
- Variables and Data Types
- Variables, Data Types
- Project: Greeting Card
- Control Flow
- Conditionals, Loops
- Project: Adventure Game
- Data Structures
- Lists, Dictionaries, Sets
- Project: To-Do List Application
- Modular Programming
- Benefits, Creating Modules, Importing Modules
- Project: Calculator
- File Operations
- File I/O, Working with Paths
- Project: Note-Keeping App
- Regular Expressions
- Pattern Matching, Data Extraction
- Project: Data Scraper
- Exception Handling
- Try/Except, Raising Exceptions
- Project: Basic Data Entry System
- Object-Oriented Programming
- Classes, Objects, Inheritance
- Project: Library Management System
- External Libraries and Visualization
- Using Libraries, Basic Data Visualization
- Project: Data Visualization with Matplotlib
- Testing and Debugging
- Unit Tests, Debugging Techniques
- Project: Testing a Shopping Cart System
- Version Control with Git
- Git Basics, GitHub, Collaboration
- Project: Collaborative Document Editor
- Final Project
- Integrating Learned Concepts
- Project: Automated Report Generation and Distribution System
- Advanced Data Types and Algorithms
- In-depth comprehension, Iterators, Generators
- Sorting and Searching Algorithms
- Project: Password Generator and Manager
- Functional Programming
- First-Class Functions, Higher-Order Functions
- Lambda Functions,
map
, filter
, reduce
- Project: Batch File Renamer
- Decorators and Closures
- Understanding and writing decorators
- Using closures for maintaining state
- Project: User Authentication System
- Context Managers and the
with
Statement
- Creating your own context managers
- Practical usage of context managers
- Project: Resource Management Tool
- Advanced Object-Oriented Programming
- MetaClasses, Abstract Base Classes
- Multiple Inheritance, Method Resolution Order
- Project: Plugin System Design
- Advanced Control Flow
- Advanced unpacking,
*args
, **kwargs
- The
itertools
and operator
modules
- Project: Command-line Calculator
- Exception Handling In-Depth
- Creating custom exception classes
- Advanced exception handling techniques
- Project: File Parser with Robust Error Handling
- File Operations and Data Serialization
- Working with binary files
- JSON, YAML, Pickle
- Project: Cross-Format Data Converter
- Database Interaction
- Python DB-API
- ORM with SQLAlchemy
- Project: Inventory Control System
- Network Programming
- Basics of the
socket
module
- Client-server application development
- Project: Peer-to-peer Chat Application
- Concurrency and Parallelism
- Threading and Multiprocessing
- AsyncIO
- Project: Asynchronous Web Scraper
- Web Development with Flask
- Flask Framework Fundamentals
- Flask Extensions
- Project: Personal Portfolio Website
- Testing and Debugging
- Unit testing with
unittest
and pytest
- Debugging and Profiling
- Project: Testing and Performance Optimization
- Automating Daily Tasks
- Scripting for File System Management
- Task Scheduling
- Project: System Backup and Task Scheduling
- Final Intermediate Project
- An integration of the concepts covered
- Developing a web service with user authentication, database interaction, API and a front-end interface
- Project: Full-stack Task Management Tool
Contributing
Contributions are always welcome! Please read CONTRIBUTING for guidelines on how to get started with contributing.
License
This project is licensed under the MIT License.
Connect and Discuss
Happy Coding! 🚀